The perf_counter () function always returns a floating time value in seconds. Returns the value (in fractions of a second) of the performance counter, that is, the clock with the highest available resolution for measuring short durations. It includes the time elapsed during sleep and is system-wide. The return value breakpoint is undefined, so only the difference between the results of successive calls is allowed. In between, we can use time.sleep () and similarly fuctions.
Code # 1: Understand the use of perf_counter .
# Python program to show time by perf_counter() from time import perf_counter # integer input from user, 2 input in single line n, m = map(int, input().split()) # Start the stopwatch / counter t1_start = perf_counter() for i in range(n): t = int(input()) # user gave input n times if t % m == 0: print(t) # Stop the stopwatch / counter t1_stop = perf_counter() print("Elapsed time:", t1_stop, t1_start) print("Elapsed time during the whole program in seconds:", t1_stop-t1_start)
Output:
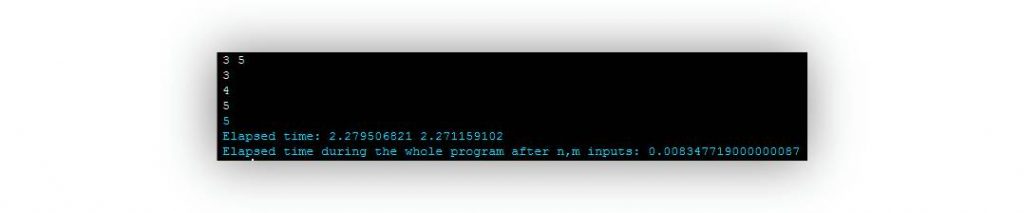
pref_counter_ns ():
It always gives an integer time value in nanoseconds. Similar to perf_counter (), but return time in nanoseconds.
Code # 2: Using perf_counter_ns and how to implement it.
# Python program to show time by # perf_counter_ns() from time import perf_counter_ns # integer input from user, 2 input in single line n, m = map(int, input().split()) # Start the stopwatch / counter t1_start = perf_counter_ns() for i in range(n): t = int(input()) # user gave input n times if t % m == 0: print(t) # Stop the stopwatch / counter t1_stop = perf_counter_ns() print("Elapsed time:", t1_stop, ’ns’, t1_start, ’ns’) print("Elapsed time during the whole program in ns after n, m inputs:", t1_stop-t1_start, ’ns’)

Compare both output from the program, since perf_counter () returns in seconds and pers_counter_ns () returns in nanoseconds.
Benefits of perf_counter ():
- perf_counter () will give you a more accurate value than time.clock () …
- From Python3.8, the time.clock () function will be removed and perf_counter will be used.
- We can calculate both float and integer values of time in seconds and nanoseconds.
Python timers
First, take a look at some of the code examples you’ll be using throughout the tutorial. Later, you will add a Python timer to this code to monitor its performance. You will also see some of the easiest ways to measure the execution time of this example.
perf counter Python
If you look at Python’s built-in time module, you’ll notice several functions that can measure time:
- monotonic()
- perf_counter()
- process_time()
- time()
Python 3.7 introduced several new functions such as thread_time() as well as nanosecond versions of all of the above functions named with the _ns suffix. For example, perf_counter_ns() is the nanosecond version of perf_counter(). Details of these functions will be discussed later. In the meantime, pay attention to what the perf_counter() documentation says:
Returns the value (in fractional seconds) of the performance counter, that is, the clock with the highest available resolution for measuring a short period of time.