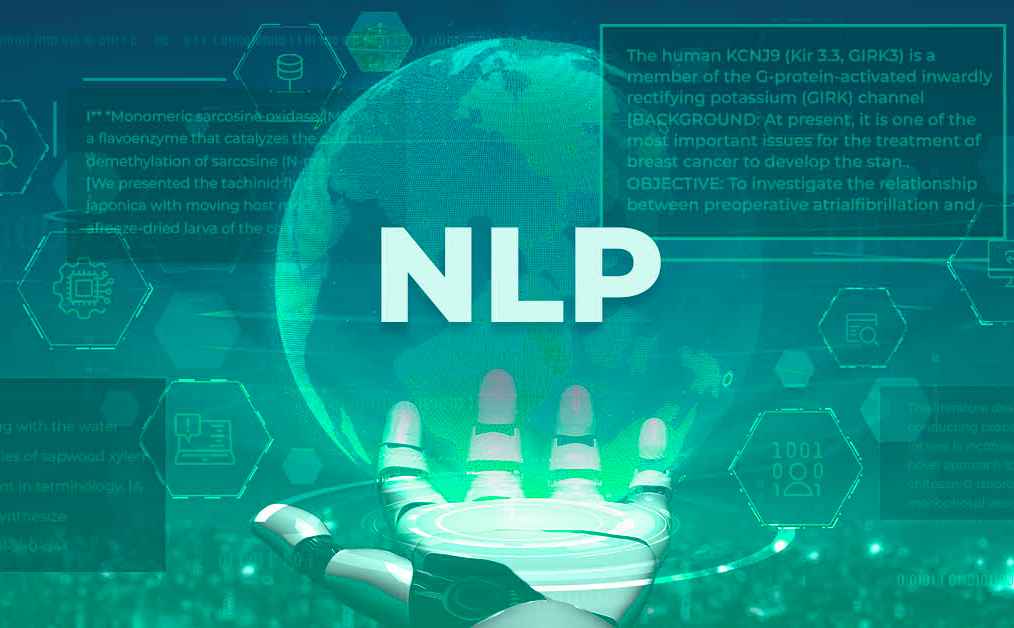
Welcome to the fascinating world of Natural Language Processing (NLP) where Python is your trusty companion in unraveling the mysteries of human language. In this comprehensive guide, we'll walk through the essential steps of NLP, from choosing the right framework to applying advanced techniques that will make you an NLP wizard.
Why NLP Matters
Natural Language Processing is not just a buzzword; it's a powerful tool that allows machines to understand and interpret human language. The importance of NLP lies in its versatility - from chatbots and virtual assistants to sentiment analysis and language translation, NLP applications are ubiquitous in today's tech landscape.
Choosing Your NLP Framework
1. NLTK - The Natural Language Toolkit
NLTK is a comprehensive library for building Python programs to work with human language data. It's a great starting point for learners and researchers due to its extensive documentation and educational resources.
pip install nltk
2. spaCy - Industrial-Strength NLP
spaCy is designed for production use, emphasizing speed and efficiency. It's a favorite among developers for its simplicity and robust performance.
pip install spacy python -m spacy download en
Basic NLP Techniques
Tokenization
Tokenization is the process of breaking text into words or phrases, and NLTK makes it a breeze:
from nltk.tokenize import word_tokenize sentence = "Python NLP is awesome!" tokens = word_tokenize(sentence) print(tokens)
Named Entity Recognition (NER) with spaCy
Named Entity Recognition identifies entities like names, locations, or organizations in a text:
import spacy nlp = spacy.load('en_core_web_sm') text = "Apple Inc. is planning to open a new store in New York." doc = nlp(text) for ent in doc.ents: print(ent.text, ent.label_)
Advanced NLP Techniques
Word Embeddings with Word2Vec
Word2Vec is a technique to represent words as vectors in a continuous vector space. The Gensim library is handy for Word2Vec implementation:
from gensim.models import Word2Vec sentences = [["python", "is", "cool"], ["natural", "language", "processing"]] model = Word2Vec(sentences, vector_size=10, window=2, min_count=1, workers=4) print(model.wv['python'])
Text Classification with BERT
Transformers by Hugging Face has revolutionized NLP with models like BERT. Text classification becomes powerful and straightforward:
from transformers import BertTokenizer, BertForSequenceClassification tokenizer = BertTokenizer.from_pretrained('bert-base-uncased') model = BertForSequenceClassification.from_pretrained('bert-base-uncased') inputs = tokenizer("Hello, how are you?", return_tensors="pt") outputs = model(**inputs)
Meet the NLP Gurus
In the vast realm of NLP, luminaries such as Yoshua Bengio, Geoffrey Hinton, and Yann LeCun have shaped the landscape with their groundbreaking contributions to deep learning and NLP.
"Natural language understanding is about converting text into a form that computers can understand."
- Yann LeCun
Frequently Asked Questions
Q: Can I use NLP for sentiment analysis?
A: Absolutely! NLP is widely used for sentiment analysis, helping businesses understand customer opinions and feedback.
Q: Are there other NLP frameworks worth exploring?
A: Yes, besides NLTK and spaCy, you might want to explore Hugging Face and TensorFlow Text for their rich set of tools and models.
Q: How can I contribute to the NLP community?
A: Join forums like OpenAI Forum and Stack Overflow, participate in NLP projects on GitHub, and attend conferences like ACL to connect with experts and enthusiasts.